Someone - C++ is faster than Python…
Show them this article…
Note - When you write same code, C++ is way more performant than most of the modern programming Languages. And you will find Python somewhere at the end of the list.
But when you implement these in some real projects, nobody cares about your code and language. What everyone cares about is the result. Many times quicker implementation is better than speed.
Let’s say you are asked to create a website. And you have only two options: Python and C++. In the end when you compare, the C++ project will be many times faster than Python. So, your server will respond and render data to clients in 0.001 sec instead of 0.01 sec. But who cares? In C++ you will end up writing code for months that can be done within a week in Python.
Now let us see matrix Multiplication
We start this holy war by implementing a matrix multiplication on two 1000x1000 sized matrix.
res_matrix = matrix1(1000x1000) multiply with matrix2(1000x1000);
Python
Python implementation code can be found here
The main code comprises of simple loops over all the elements -
for i in range(n):
for j in range(n):
for k in range(n):
res_matrix[i][j] += matrix1[i][k] * matrix2[k][j];
How long did it take? 141.073768 sec ……….(for n = 1000 )
Rewriting the same code in C++
You can find the implementation here .
The main code comprises of simple loops over all the elements -
for(int i = 0; i < n; i++){
for(int j = 0; j < n; j++){
for(int k = 0; k < n; k++){
res_matrix[i][j] += matrix1[i][k] * matrix2[k][j];
}
}
}
With 1000 by 1000, it did it in - 9.761765 sec Which is around 15x times faster than Python.
Why so?
C++ is compiled whereas python is interpreted.
Interpreted vs Compiled Programming Languages
Every program is a set of instructions. Compilers and interpreters take human-readable code and convert it into machine code. In a compiled language, the code is directly converted to machine code that the processor can execute. As a result, they tend to be faster and more efficient. Whereas in an interpreted language the source code is translated using a different program (also known as interpreter) which reads and executes the code. Interpreters run through a program line by line and execute each command, but in Compiled languages you need to manually compile whole code each time you make a change.
Compiled languages also give the developer more control over hardware aspects, like memory management and CPU usage.
Let’s see a magic-
If we change the loop order in above C++ implementation, it keeps the correctness while the speed increases
for(int i = 0; i < n; i++){
for(int k = 0; k < n; k++){
for(int j = 0; j < n; j++){
res_matrix[i][j] += matrix1[i][k] * matrix2[k][j];
}
}
}
for n = 1000 this code took 7.491608 sec
for large value of n this can be 5x times faster than above C++ implementation. Here Speed increase because cache hit increases. For first implementation cache miss rate was really high!
But if we change the loop order in Python Code. There won’t be any time difference.
We can further optimize the C++ programs to run in less than 1 sec with the help of compiler, changing the algorithm and many other things.
Conclusion
Wait… nobody is writing logic in python because “Libraries included”
Sure, these libraries are written in C++, But who cares?
Multiplying Matrices with Numpy
import numpy as np
res_matrix = np.matmul(matrix1, matrix2)
And… \ the time is…
…
…
0.112477 sec
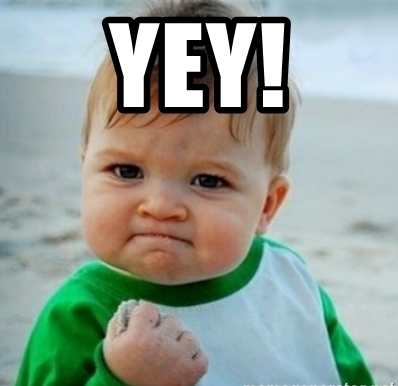
Can you believe that?
Some excuses… :)
Yep. Numpy is written in C++.
But come on! You will never be implementing the same advanced algorithm that is in Numpy yourself if you are writing in C++.
And sure, you can do so much with C++ you cannot do with Python.